What is a Structure
Structure is user defined data type which is used to store heterogeneous data under unique name. Keyword 'struct' is used to declare structure.
The variables which are declared inside the structure are called as 'members of structure'.
Why Use StructuresWe have seen earlier how ordinary variables can hold one piece of information and how arrays can hold a number of pieces of information of the same data type.
These two data types can handle a great variety of situations. But quite often we deal with entities that are collection of dissimilar data types.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
(a)Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
(b)Use a structure variable.
The first approach become difficult and complex.
C provides a special data type—the structure.
A structure contains a number of data types grouped together. These data types may or may not be of the same type.
Syntax:
struct structure-name
{
- - - - - - - - - - -
- - - - - - - - - - -
}struct_variable;
Example :
struct emp_info
{
char emp_id[10];
char nm[100];
float sal;
}emp;
Note :
1. Structure is always terminated with semicolon (;).
2. Structure name as emp_info can be later used to declare structure variables of its type in a program.
Instances of Structure :
Instances of structure can be created in two ways as,
Instance 1:struct emp_info
{
char emp_id[10];
char name[100];
float salary;
}emp;
Instance 2:
struct emp_info
{
char emp_id[10];
char name[100];
float salary;
};
struct emp_info emp;
In above example, emp_info is a simple structure which consists of stucture members as Employee ID(emp_id), Employee Name(nm), Employee Salary(sal).
Aceessing Structure Members :
Structure members can be accessed using member operator '.' . It is also called as 'dot operator' or 'period operator'.
structure_var.member;Example :
#include <stdio.h>
#include <conio.h>
struct comp_info
{
char name[100];
char addr[100];
}info;
void main()
{
clrscr();
printf("\n Enter Company Name : ");
gets(info.name);
printf("\n Enter Address : ");
gets(info.address);
printf("\n\n Company Name : %s",info.name);
printf("\n\n Address : %s",info.address);
getch();
}
Note : gets()function is used to enter the input. The advantage is that it takes the input with space character.
info.name---'dot' operator is used to access the name
info.address---'dot' operator is used to access the address
Output :
Enter Company Name : Software Solutions
Enter Address : Chandigarh
Company Name : Software Solutions
Address : Chandigarh
Array in Structures :
Sometimes, it is necessary to use structure members with array.
Example :
#include <stdio.h>
#include <conio.h>
struct result
{
int rno;
int mrks[5]; //array is used
char name;
}res;
void main()
{
int i,total;
clrscr();
total = 0;
printf("\n\t Enter Roll Number : ");
scanf("%d",&res.rno);
printf("\n\t Enter Marks of 3 Subjects : ");
for(i=0;i<3;i++)
{
scanf("%d",&res.mrks[i]); //array is used
total = total + res.mrks[i];
}
printf("\n\n\t Roll Number : %d",res.rno);
printf("\n\n\t Marks are :");
for(i=0;i<3;i++)
{
printf(" %d",res.mrks[i]);
}
printf("\n\n\t Total is : %d",total);
getch();
}
Output :
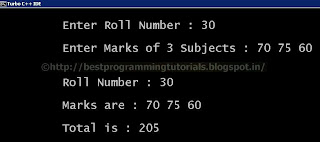
Structures with array :
We can create structures with array for ease of operations in case of getting multiple same fields.
Example :#include <stdio.h>
#include <conio.h>
struct result
{
int rno;
int mrks[5]; //array is used
char nm;
}res;
void main()
{
int i,total;
clrscr();
total = 0;
printf("\n\t Enter Roll Number : ");
scanf("%d",&res.rno);
printf("\n\t Enter Marks of 3 Subjects : ");
for(i=0;i<3;i++)
{
scanf("%d",&res.mrks[i]); //array is used
total = total + res.mrks[i];
}
printf("\n\n\t Roll Number : %d",res.rno);
printf("\n\n\t Marks are :");
for(i=0;i<3;i++)
{
printf(" %d",res.mrks[i]);
}
printf("\n\n\t Total is : %d",total);
getch();
}
Output :
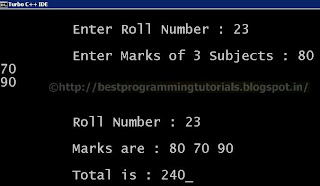
Structure within a structure(Nesting of structure) :
Structures can be used as structures within structures. It is also called as 'nesting of structures'.
Syntax:
struct structure_nm
{
element 1;
element 2;
- - - - - - - - - - -
- - - - - - - - - - -
element n;
struct structure_nm
{
element 1;
element 2;
- - - - - - - - - - -
- - - - - - - - - - -
element n;
}inner_struct_var;
}outer_struct_var;
Example :
struct stud_Res
{
int rno;
char nm[50];
char std[10];
struct stud_subj
{
char subjnm[30];
int marks;
}subj;
}result;
In above example, the structure stud_Res consists of stud_subj which itself is a structure with two members. Structure stud_Res is called as 'outer structure' while stud_subj is called as 'inner structure.' The members which are inside the inner structure can be accessed as follow :
result.subj.subjnm
result.subj.marks
Example :
#include <stdio.h>
#include <conio.h>
struct stud_Res
{
int rno;
char std[10];
struct stud_Marks
{
char subj_nm[30];
int subj_mark;
}marks;
}result;
void main()
{
clrscr();
printf("\n\t Enter Roll Number : ");
scanf("%d",&result.rno);
printf("\n\t Enter Standard : ");
scanf("%s",result.std);
printf("\n\t Enter Subject Code : ");
scanf("%s",result.marks.subj_nm);
printf("\n\t Enter Marks : ");
scanf("%d",&result.marks.subj_mark);
printf("\n\n\t Roll Number : %d",result.rno);
printf("\n\n\t Standard : %s",result.std);
printf("\n\n\tSubject Code : %s",result.marks.subj_nm);
printf("\n\n\t Marks : %d",result.marks.subj_mark);
getch();
}
Output :
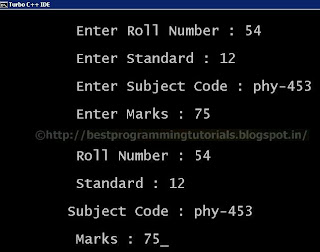
Summary :
(a)A structure is usually used when we wish to store dissimilar data together.
(b)Structure elements can be accessed through a structure variable using a dot (.)
operator.
(c)Structure elements can be accessed through a pointer to a structure using the
arrow (->) operator.
(d)All elements of one structure variable can be assigned to another structure variable using
the assignment (=) operator.
(e)It is possible to pass a structure variable to a function either by value or by address.
(f)It is possible to create an array of structures.
Prev NEXT
0 comments:
Post a Comment