What is an Array
An array is a collection of similar elements. These similar elements could be all ints, or all floats, or all chars, etc.
For understanding the arrays properly, let us consider the following program:
main( )
{
int x ;
x = 5 ;
x = 10 ;
printf ( "\nx = %d", x ) ;
}
No doubt, this program will print the value of x as 10. Why so? Because when a value 10 is assigned to x, the earlier value of x, i.e. 5, is lost. Thus, ordinary variables (the ones which we have used so far) are capable of holding only one value at a time (as in the above example). However, there are situations in which we would want to store more than one value at a time in a single variable.
For example, suppose we wish to arrange the percentage marks obtained by 100 students in ascending order. In such a case we have two options to store these marks in memory:
(a) Construct 100 variables to store percentage marks obtained by 100 different students, i.e. each variable containing one student’s marks.
(b)Construct one variable (called array or subscripted variable) capable of storing or holding all the hundred values.
Obviously, the second alternative is better. A simple reason for this is, it would be much easier to handle one variable than handling 100 different variables.
Moreover, there are certain logics that cannot be dealt with, without the use of an array. Now a formal definition of an array—An array is a collective name given to a group of ‘similar quantities’. These similar quantities could be percentage marks of 100 students, or salaries of 300 employees, or ages of 50 employees. What is important is that the quantities must be ‘similar’. Each member in the group is referred to by its position in the group. For example, assume the following group of numbers, which represent percentage marks obtained by five students.
per = { 48, 88, 34, 23, 96 }
If we want to refer to the second number of the group, the usual notation used is per[2] which have value 88. Similarly per[0] will denote the first element that is 48.This is because in C the counting of elements begins with 0 and not with 1.
A Simple Program Using Array
Let us try to write a program to find average marks obtained by a
class of 30 students in a test.
main( )
{
int avg, sum = 0 ;
int i ;
int marks[30] ; /* array declaration */
for ( i = 0 ; i <= 29 ; i++ )
{
printf ( "\nEnter marks " ) ;
scanf ( "%d", &marks[i] ) ; /* store data in array */
}
for ( i = 0 ; i <= 29 ; i++ )
sum = sum + marks[i] ; /* read data from an array*/
avg = sum / 30 ;
printf ( "\nAverage marks = %d", avg ) ;
}
There is a lot of new material in this program, so let us take it apart
slowly.
Array Declaration
To begin with, like other variables an array needs to be declared so that the compiler will know what kind of an array and how large an array we want. In our program we have done this with the statement:
int marks[30] ;
- Here, int specifies the type of the variable, just as it does with ordinary variables and the word marks specifies the name of the variable.
- The number 30 tells how many elements of the type int will be in our array. This number is often called the ‘dimension’ of the array.
- The bracket ( [ ] ) tells the compiler that we are dealing with an array.
- All the array elements are numbered, starting with 0. Thus, marks[2] is not the second element of the array, but the third.
- In our program we are using the variable i as a subscript to refer to various elements of the array. This variable can take different values and hence can refer to the different elements in the array in turn. This ability to use variables as subscripts is what makes arrays so useful.
Entering Data into an Array
Here is the section of code that places data into an array:
for ( i = 0 ; i <= 29 ; i++ )
{
printf ( "\nEnter marks " ) ;
scanf ( "%d", &marks[i] ) ;
}
The for loop causes the process of asking for and receiving a student’s marks from the user to be repeated 30 times.
The first time through the loop, i has a value 0, so the scanf( ) function will cause the value typed to be stored in the array element marks[0], the first element of the array. This process will be repeated until ibecomes 29. This is last time through the loop, which is a good thing, because there is no array element like marks[30].
In scanf( ) function, we have used the “address of” operator (&) on the element marks[i] of the array, just as we have used it earlier on other variables (&rate, for example).
In so doing, we are passing the address of this particular array element to the scanf( ) function, rather than its value; which is what scanf( ) requires.
Reading Data from an Array
The balance of the program reads the data back out of the array and uses it to calculate the average. The for loop is much the same, but now the body of the loop causes each student’s marks to be added to a running total stored in a variable called sum. When all the marks have been added up, the result is divided by 30, the number of students, to get the average.
for ( i = 0 ; i <= 29 ; i++ )
sum = sum + marks[i] ;
avg = sum / 30 ;
printf ( "\nAverage marks = %d", avg ) ;
To fix our ideas, let us revise whatever we have learnt about arrays:
(a)An array is a collection of similar elements.
(b)The first element in the array is numbered 0, so the last element is 1 less than the size of the array.
(c)An array is also known as a subscripted variable.
(d)Before using an array its type and dimension must be
declared.
Types of Array
An array is of two types :
- One/Single dimensional array
- Two dimensional array
The array which is used to represent and store data in a linear form is called as 'single or one dimensional array.'
Syntax:[size];
Example:
int a[3] = {2, 3, 5};
char ch[20] = "Ashish" ;
float stax[3] = {5003.23, 1940.32, 123.20} ;
Total Size (in Bytes):
total size = length of array * size of data type
In above example, a is an array of type integer which has storage size of 3 elements. The total size would be 3 * 2 = 6 bytes.
Memory Allocation :
Fig : Memory allocation for one dimensional array
Example :
#include <stdio.h>#include <conio.h>
void main()
{
int a[3], i;;
clrscr();
printf("\n\t Enter three numbers : ");
for(i=0; i<3; i++)
{
scanf("%d", &a[i]); // read array
}
printf("\n\n\t Numbers are : ");
for(i=0; i<3; i++)
{
printf("\t %d", a[i]); // print array
}
getch();
}
Output :
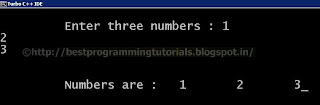
Two dimensional array
So far we have explored arrays with only one dimension. It is also possible for arrays to have two or more dimensions. The twodimensional array is also called a matrix. Here is a sample program that stores roll number and marks obtained by a student side by side in a matrix.
main( )
{
int stud[4][2] ;
int i, j ;
for ( i = 0 ; i <= 3 ; i++ )
{
printf ( "\n Enter roll no. and marks" ) ;
scanf ( "%d %d", &stud[i][0], &stud[i][1] ) ;
}
for ( i = 0 ; i <= 3 ; i++ )
printf ( "\n%d %d", stud[i][0], stud[i][1] ) ;
}
There are two parts to the program—in the first part through a for loop we read in the values of roll no. and marks, whereas, in second part through another for loop we print out these values.
Look at the scanf( ) statement used in the first for loop:
scanf ( "%d %d", &stud[i][0], &stud[i][1] ) ;
In stud[i][0] and stud[i][1] the first subscript of the variable stud, is row number which changes for every student. The second subscript tells which of the two columns are we talking about—
the zeroth column which contains the roll no. or the first column which contains the marks. Remember the counting of rows and columns begin with zero. The complete array arrangement is shown below.
col. no. 0 col. no. 1
row no. 0 1234 56
row no. 1 1212 33
row no. 2 1434 80
row no. 3 1312 78
Thus, 1234 is stored in stud[0][0], 56 is stored in stud[0][1] and so on. The above arrangement highlights the fact that a two dimensional array is nothing but a collection of a number of one dimensional arrays placed one below the other.
In our sample program the array elements have been stored rowwise and accessed rowwise. However, you can access the array elements columnwise as well.
The following syntax is used to represent two dimensional array.
Syntax:
Example:
#include <stdio.h>
#include <conio.h>
void main()
{
int a[3][3], i, j;
clrscr();
printf("\n\t Enter matrix of 3*3 : ");
for(i=0; i<3; i++)
{
for(j=0; j<3; j++)
{
scanf("%d",&a[i][j]); //read 3*3 array
}
}
printf("\n\t Matrix is : \n");
for(i=0; i<3; i++)
{
for(j=0; j<3; j++)
{
printf("\t %d",a[i][j]); //print 3*3 array
}
printf("\n");
}
getch();
}
Output :
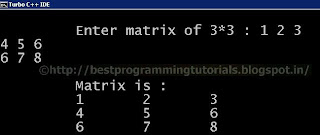
Limitations of two dimensional array :
- We cannot delete any element from an array.
- If we dont know that how many elements have to be stored in a memory in advance, then there will be memory wastage if large array size is specified.
(a)An array is similar to an ordinary variable except that it can store multiple elements of similar type.
(b)Compiler doesn’t perform bounds checking on an array.
(c)The array variable acts as a pointer to the zeroth element of the array.
In a 1-D array, zeroth element is a single value, whereas, in a 2-D array this element is a 1-D array.
(d)On incrementing a pointer it points to the next location of its type.
(e)Array elements are stored in contiguous memory locations and so they can be accessed using pointers.
Prev NEXT
0 comments:
Post a Comment