C Switch statement
When we use nested if-else statement to check more than 1 conditions then the complexity of a program increases in case of a lot of conditions. Thus, the program is difficult to read and maintain. So to overcome this problem, C provides 'switch case'.
Switch case checks the value of a expression against a case values, if condition matches the case values then the control is transferred to that point.
Syntax:switch(expression)
{
case expr1:
statements;
break;
caseexpr2:
statements;
break;
................
................
case exprn:
statements;
break;
default:
statements;
}
In above syntax, switch, case, break are keywords.expr1, expr2 are known as 'case labels.'Statements inside case expression need not to be closed in braces.
Break statement causes an exit from switch statement.Default case is optional case. When neither any match found, it executes.
Example :
#include <conio.h>
void main()
{
int no;
clrscr();
printf("\n Enter any number from 1 to 3 :");
scanf("%d",&no);
switch(no)
{
case 1:
printf("\n\n It is 1 !");
break;
case 2:
printf("\n\n It is 2 !");
break;
case 3:
printf("\n\n It is 3 !");
break;
default:
printf("\n\n Invalid number !");
}
getch();
}
Output :
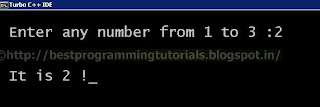
Note:
break should be placed at the end of the case otherwise unexpected output will be display.
Example:
#include <stdio.h>
#include <conio.h>
{
int no;
clrscr();
printf("\n Enter any number from 1 to 3 :");
scanf("%d",&no);
switch(no)
{
case 1:
printf("\n\n It is 1 !");
case 2:
printf("\n\n It is 2 !");
case 3:
printf("\n\n It is 3 !");
default:
printf("\n\n Invalid number !");
}
getch();
}
Output :
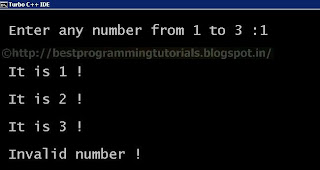
Description :
Since there is no break statement at the end of case , when the user enter 1 first case is matched but there is not any break statement at the end so all other case including default will be executed too.
Prev NEXT
0 comments:
Post a Comment